NumPy Introduction 2
function
1 | import numpy as np |
A faster way
1 | big_array = np.random.randint(1, 100, size=1000000) |
prove:
1 | print(compute_reciprocals(values)) |
*arange calculation
1 | np.arange(5) / np.arange(1, 6) #[0 1 2 3 4] [1 2 3 4 5] |
1 | x = np.arange(9).reshape((3, 3)) |
add,subtract,multiply,divide
1 | x = np.arange(4) |
others
1 | print("-x = ", -x) |
get them together
1 | -(0.5*x + 1) ** 2 |
.add()
1 | np.add(x, 2) #add 2 to every value |
运算符 | 对应的通用函数 | 描述 | example |
---|---|---|---|
+ | np.add | 加法运算 | (即 1 + 1 = 2) |
- | np.subtract | 减法运算 | (即 3 - 2 = 1) |
- | np.negative | 负数运算 | (即 -2) |
* | np.multiply | 乘法运算 | (即 2 * 3 = 6) |
/ | np.divide | 除法运算 | (即 3 / 2 = 1.5) |
// | np.floor_divide | 地板除法运算 | (flfloor division,即 3 // 2 = 1) |
** | np.power | 指数运算 | (即 2 ** 3 = 8) |
% | np.mod | 模 / 余数 | (即 9 % 4 = 1) |
abs() absolute()
1 | x = np.array([-2, -1, 0, 1, 2]) |
1 | theta = np.linspace(0, np.pi, 3) |
reverse:
1 | x = [-1, 0, 1] |
log and ln
1 | x = [1, 2, 3] |
more advanced
1 | x = np.arange(5) #from 0 to 4 |
reduce()
1 | x = np.arange(1, 6) #[1 2 3 4 5] |
outer()
1 | x = np.arange(1, 6) |
sum()
1 | L = np.random.random(100) |
*faster way:
1 | big_array = np.random.rand(1000000) |
python has its own function min and max, but numpy’s is faster.
1 | np.min(big_array), np.max(big_array) |
1 | M = np.random.random((3, 4)) |
example: (USA presidents’ height)
data: (.csv)
order,name,height(cm)
1,George Washington,189
2,John Adams,170
3,Thomas Jefferson,189
1 | import numpy as np |
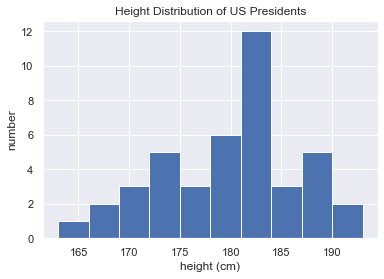